In programming, variables are essential as they allow developers to store, manipulate, and retrieve data efficiently. In PHP, variables play a crucial role in managing information dynamically. Whether you are creating a simple script or a complex web application, understanding how PHP variables work is fundamental to writing effective code.
In this guide, we will explore PHP variables in depth, covering their syntax, data types, scope, and best practices for naming conventions. By the end, you’ll have a solid foundation for using variables in your PHP projects.
Declaring and Assigning PHP Variables
In PHP, variables are declared using the $
symbol followed by the variable name. Unlike some other languages, PHP does not require explicit type declarations. The value assigned to a variable determines its data type dynamically.
Example:
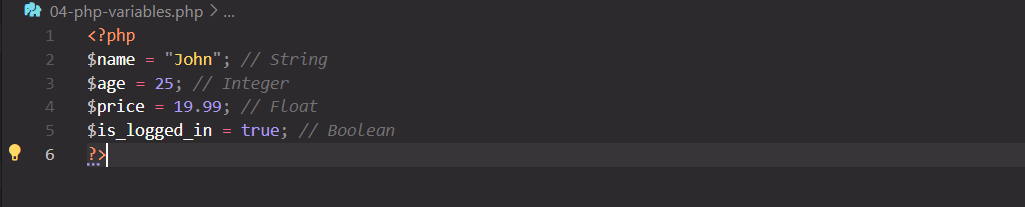
PHP Variable Naming Conventions
When naming PHP variables, follow these best practices:
- Variable names must start with a letter or an underscore (
_
). - They cannot start with a number.
- They are case-sensitive (
$name
and$Name
are different variables). - Use meaningful names for better readability (
$userName
instead of$a
). - Follow camelCase or snake_case naming conventions (
$firstName
or$first_name
).
[code_editor]
PHP Data Types
Data Type | Example | Meaning |
---|---|---|
String | "Hello" | Text |
Integer | 100 | Whole number |
Float | 5.75 | Decimal number |
Boolean | true or false | Yes/No values |
Array | ["Apple", "Banana"] | List of values |
Object | new Car(); | Custom structure |
NULL | NULL | No value |
PHP supports several data types that define the nature of stored values.
1. String
A string is just a piece of text (letters, words, sentences).
<?php
$greeting = "Hello, World!";
echo $greeting;
?>
2. Integer
An integer is a number without a decimal (like 1, 10, -5).
<?php
$age = 30;
echo $age;
?>
3. Float (Double)
A float is a number with a decimal (like 3.14, 10.5).
<?php
$price = 99.99;
echo $price;
?>
4. Boolean
A boolean is either true or false (used for yes/no, on/off type answers).
<?php
$is_active = true;
?>
5. Array
An array holds multiple values (like a shopping list).
<?php
$fruits = array("Apple", "Banana", "Orange");
echo $fruits[0]; // Outputs Apple
?>
6. Object
An object is like a blueprint that holds different types of data together.
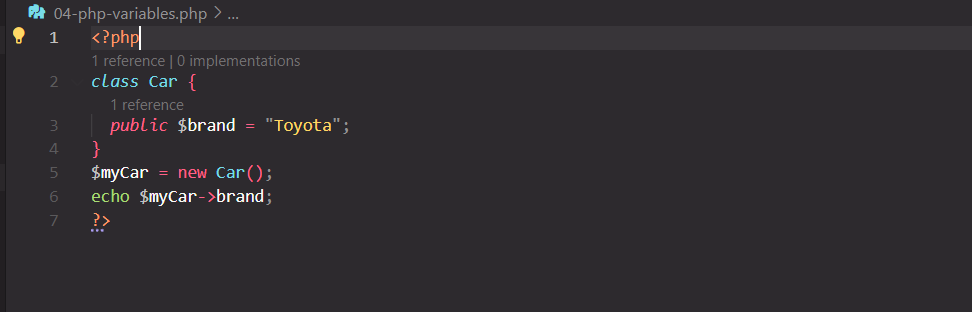
7. NULL
Represents a variable with no value.
<?php
$var = NULL;
echo $var;
?>
PHP Variable Scope
Variable scope defines where a variable can be accessed in a PHP script. PHP supports three main types of variable scope:
1. Local Scope
A variable declared inside a function is accessible only within that function.
<?php
function myFunction() {
$message = "Hello!";
echo $message;
}
myFunction();
// echo $message; // This will cause an error
?>
2. Global Scope
A variable declared outside a function can be accessed using the global
keyword inside the function.
<?php
$globalVar = "I am global";
function testGlobal() {
global $globalVar;
echo $globalVar;
}
testGlobal();
?>
3. Static Scope
A variable declared as static
retains its value across function calls.
<?php
function counter() {
static $count = 0;
$count++;
echo $count;
}
counter(); // Outputs 1
counter(); // Outputs 2
?>
Dynamic Variables in PHP
Dynamic variables allow variable names to be assigned dynamically using another variable.
<?php
$varName = "greeting";
$greeting = "Hello, World!";
echo $$varName; // Outputs Hello, World!
?>
Use Cases and Warnings:
- Useful for creating dynamic configurations.
- Can be risky if improperly handled, leading to security vulnerabilities.
Conclusion
Understanding PHP variables is crucial for writing efficient and readable code. In this guide, we explored variable declaration, naming conventions, data types, scope, and dynamic variables. With these concepts, you can start working with PHP variables confidently in your projects.
FAQs
What are PHP variables?
PHP variables store and manipulate data dynamically. They start with a $
symbol.
What is variable scope in PHP?
Variable scope determines where a variable can be accessed. PHP supports local, global, and static scopes.
What are the PHP data types?
PHP supports strings, integers, floats, booleans, arrays, objects, and NULL.
How do you assign a variable in PHP?
You assign a variable using the =
operator, e.g., $name = "John";
.
What are dynamic variables in PHP?
Dynamic variables allow you to use variable names stored in other variables.
[mcq_quiz id=”html”]