PHP (Hypertext Preprocessor) is a popular server-side scripting language for web development. Its syntax is easy to learn and integrates seamlessly with HTML, making it an excellent choice for beginners. Understanding PHP syntax is the first step toward building dynamic web applications. This guide will explore PHP syntax with examples, cheat sheets, and a syntax checker to help you write clean and error-free code.
Basic PHP Syntax
To use PHP in a file, it must be enclosed within PHP tags:

Every PHP script starts with <?php
and ends with ?>
. Inside these tags, you can write PHP code that interacts with databases, handles forms, or performs calculations.
Example of a Simple PHP Script:
<?php
echo "Hello, World!";
?>
Output: Hello, World!
Embedding PHP in HTML
PHP can be embedded directly into HTML. Here’s an example:
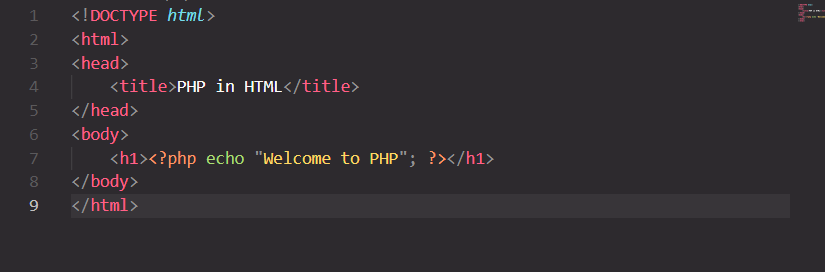
PHP Syntax List and Examples
1. Variables and Data Types
PHP variables start with a $
sign and do not require explicit data type declarations.
<?php
$txt = "Hello, PHP!";
$number = 100;
$floatNum = 10.5;
$boolean = true;
?>
2. PHP Comments
Comments are ignored by the PHP interpreter but help developers understand the code.
// Single-line comment
# Another single-line comment
/* Multi-line comment */
3. PHP Operators
PHP supports arithmetic, assignment, comparison, and logical operators.
<?php
$a = 10;
$b = 5;
echo $a + $b; // Outputs 15
?>
4. Control Structures
Conditional Statements
<?php
$age = 20;
if ($age >= 18) {
echo "You are eligible to vote.";
} else {
echo "You are not eligible to vote.";
}
?>
Loops in PHP
<?php
for ($i = 1; $i <= 5; $i++) {
echo "Number: $i <br>";
}
?>
PHP Syntax Checker and Cheat Sheet
A PHP syntax checker helps detect errors in your code. Use tools like:
For a quick PHP syntax reference, check out this PHP Syntax Cheat Sheet.
Common PHP Syntax Errors and How to Fix Them
- Missing Semicolon: Every PHP statement should end with
;
- Mismatched Quotes: Ensure strings are enclosed correctly in single (
'
) or double ("
) quotes. - Unclosed Brackets: Always close
{}
,()
, and[]
properly.
Conclusion
Understanding PHP syntax is essential for developing dynamic web applications. From variables to loops and functions, mastering these basics will help you build robust web applications. Keep practicing and use PHP syntax checkers to refine your skills.
Frequently Asked Questions (FAQs)
What is the basic syntax of PHP?
PHP scripts begin with <?php
and end with ?>
. Statements are written inside these tags and executed on the server.
Is PHP syntax easy?
Yes, PHP syntax is beginner-friendly and similar to C, Java, and JavaScript, making it easier to learn.
What is included in PHP syntax?
PHP syntax includes variables, data types, operators, functions, control structures, and error handling mechanisms.
What is PHP structure?
PHP structure consists of opening and closing tags, statements, loops, functions, and database interactions.
What is PHP basics?
The basics include variables, arrays, loops, conditionals, functions, and form handling.
What is the basic of syntax?
Basic syntax includes statements ending in semicolons, correct use of brackets, and function definitions.
What is PHP syntax error?
A syntax error occurs when PHP fails to understand the code due to missing semicolons, unclosed brackets, or incorrect syntax.
What does syntax mean in PHP?
Syntax refers to the rules that define how PHP code should be written and structured.
What is the full form of PHP syntax?
PHP stands for “Hypertext Preprocessor,” and syntax refers to its grammatical structure.
What is object syntax in PHP?
Object syntax involves defining classes and creating objects:
<?php
class Car {
public $brand;
function setBrand($brand) {
$this->brand = $brand;
}
}
$myCar = new Car();
$myCar->setBrand("Toyota");
echo $myCar->brand;
?>
What is basic syntax in HTML?
HTML syntax consists of tags enclosed in < >
, such as <html>
, <head>
, <body>
, and <p>
.